Use inheritance for my first program (cpp)
Who is the intruder ?
Find the most revelant intruder
Our program works pretty well but let's question it with the words: pr2, kevin et bob
You should have this:
We can represent this part of the ontology as follow:
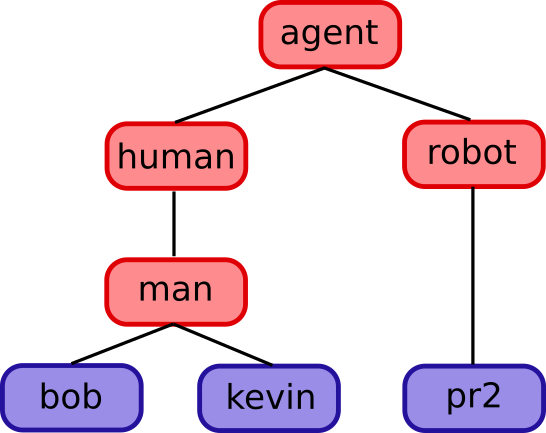
The result is not false but only one of these two answers would be enough given that one induces the other. We will use ontologenius to try to give only the most relevant answer in such cases.
To do this we will use a new function: getDown
As you can imagine, the getDown function is the exact opposite of the getUp function, meaning that it returns the concepts inheriting from the one passed in the parameter of the function.
We will use this function on classes because no concept can inherit an individual but especially because our goal is to find the reasons (which are classes) that overlap.
So we notice that we are going to look for the object classes of the ontology manipulator instead of the object individuals.
The getUp function is available for individuals, classes, and properties while the getDown function is only available for classes and properties.
We can call our refining function in our main function just after the function to find intruders
Re-interrogating our program with the words pr2, kevin, and bob, we now have the following result:
If you want this to be the concept "human" that explains the intruder, you just have to modify the algorithm above using the getUp function rather than the getDown function. It is your turn!